Adding empty element to declared container without declaring type of element
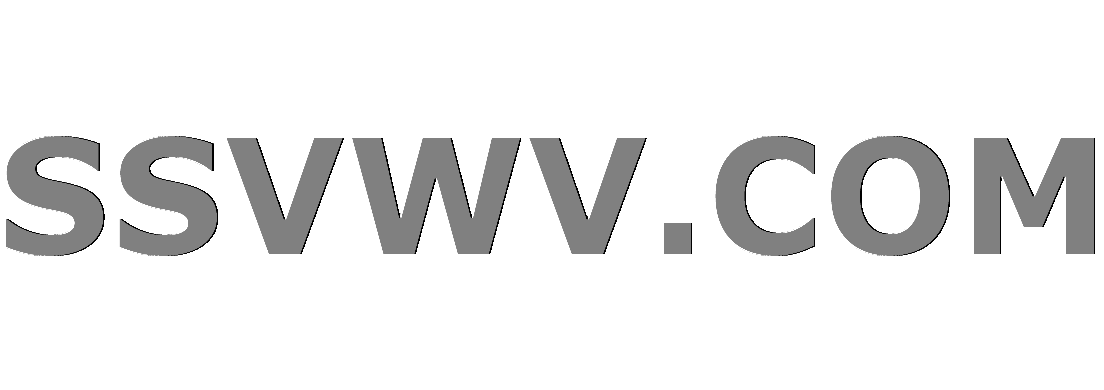
Multi tool use
When we use a complicated container in C++, like
std::vector<std::map<std::string, std::set<std::string>>> table;
The only way to add an empty map (which may represent a row or column) is to initialize a new element and push it back. For example with
table.push_back(std::map<std::string, std::set<std::string>>());
Is there any way to avoid redeclaring the type, and just adding the correct typed element?
c++ c++11
add a comment |
When we use a complicated container in C++, like
std::vector<std::map<std::string, std::set<std::string>>> table;
The only way to add an empty map (which may represent a row or column) is to initialize a new element and push it back. For example with
table.push_back(std::map<std::string, std::set<std::string>>());
Is there any way to avoid redeclaring the type, and just adding the correct typed element?
c++ c++11
add a comment |
When we use a complicated container in C++, like
std::vector<std::map<std::string, std::set<std::string>>> table;
The only way to add an empty map (which may represent a row or column) is to initialize a new element and push it back. For example with
table.push_back(std::map<std::string, std::set<std::string>>());
Is there any way to avoid redeclaring the type, and just adding the correct typed element?
c++ c++11
When we use a complicated container in C++, like
std::vector<std::map<std::string, std::set<std::string>>> table;
The only way to add an empty map (which may represent a row or column) is to initialize a new element and push it back. For example with
table.push_back(std::map<std::string, std::set<std::string>>());
Is there any way to avoid redeclaring the type, and just adding the correct typed element?
c++ c++11
c++ c++11
edited Mar 25 at 10:41


Mohammad Usman
21.4k134758
21.4k134758
asked Mar 25 at 6:35
VineetVineet
354411
354411
add a comment |
add a comment |
5 Answers
5
active
oldest
votes
From CLion's IntelliSense, I later found that one useful method is emplace_back()
. This constructs a new object of correct type and adds it to the end of the vector.
table.emplace_back();
add a comment |
You can take advantage of copy-list-initialization (since C++11) and just write
table.push_back({});
add a comment |
Before C++11 sometimes I use x.resize(x.size()+1)
, in C++11 or later you can use x.push_back({})
.
add a comment |
Though the other answers are correct, I will add that if you couldn't take that approach, you could have benefitted from declaring some type aliases to shorten that container type name.
I can of course only guess at the logical meaning of your containers, which is another thing that this fixes!
using PhilosopherNameType = std::string;
using NeighboursType = std::set<PhilosopherNameType>;
using NeighbourMapType = std::map<PhilosopherNameType, NeighboursType>;
std::vector<NeighbourMapType> table;
table.push_back(NeighbourMapType());
I mention this because you can likely still benefit from this in other places.
2
agreed. Along the same lines are the types that the STL gives you (vector::value_type iirc)
– sudo rm -rf slash
Mar 26 at 8:01
add a comment |
As a example:
vector<int> arr;
arr.push_back(1);
arr.push_back(2);
To use it, you should:
include
and also, either use std::vector
in your code or add
using std::vector;
or
using namespace std;
after the #include
line.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55332359%2fadding-empty-element-to-declared-container-without-declaring-type-of-element%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
From CLion's IntelliSense, I later found that one useful method is emplace_back()
. This constructs a new object of correct type and adds it to the end of the vector.
table.emplace_back();
add a comment |
From CLion's IntelliSense, I later found that one useful method is emplace_back()
. This constructs a new object of correct type and adds it to the end of the vector.
table.emplace_back();
add a comment |
From CLion's IntelliSense, I later found that one useful method is emplace_back()
. This constructs a new object of correct type and adds it to the end of the vector.
table.emplace_back();
From CLion's IntelliSense, I later found that one useful method is emplace_back()
. This constructs a new object of correct type and adds it to the end of the vector.
table.emplace_back();
edited Mar 25 at 7:12
answered Mar 25 at 6:51
VineetVineet
354411
354411
add a comment |
add a comment |
You can take advantage of copy-list-initialization (since C++11) and just write
table.push_back({});
add a comment |
You can take advantage of copy-list-initialization (since C++11) and just write
table.push_back({});
add a comment |
You can take advantage of copy-list-initialization (since C++11) and just write
table.push_back({});
You can take advantage of copy-list-initialization (since C++11) and just write
table.push_back({});
edited Mar 25 at 6:46
answered Mar 25 at 6:37
songyuanyaosongyuanyao
93.8k11182249
93.8k11182249
add a comment |
add a comment |
Before C++11 sometimes I use x.resize(x.size()+1)
, in C++11 or later you can use x.push_back({})
.
add a comment |
Before C++11 sometimes I use x.resize(x.size()+1)
, in C++11 or later you can use x.push_back({})
.
add a comment |
Before C++11 sometimes I use x.resize(x.size()+1)
, in C++11 or later you can use x.push_back({})
.
Before C++11 sometimes I use x.resize(x.size()+1)
, in C++11 or later you can use x.push_back({})
.
answered Mar 25 at 6:49
65026502
87.6k13115218
87.6k13115218
add a comment |
add a comment |
Though the other answers are correct, I will add that if you couldn't take that approach, you could have benefitted from declaring some type aliases to shorten that container type name.
I can of course only guess at the logical meaning of your containers, which is another thing that this fixes!
using PhilosopherNameType = std::string;
using NeighboursType = std::set<PhilosopherNameType>;
using NeighbourMapType = std::map<PhilosopherNameType, NeighboursType>;
std::vector<NeighbourMapType> table;
table.push_back(NeighbourMapType());
I mention this because you can likely still benefit from this in other places.
2
agreed. Along the same lines are the types that the STL gives you (vector::value_type iirc)
– sudo rm -rf slash
Mar 26 at 8:01
add a comment |
Though the other answers are correct, I will add that if you couldn't take that approach, you could have benefitted from declaring some type aliases to shorten that container type name.
I can of course only guess at the logical meaning of your containers, which is another thing that this fixes!
using PhilosopherNameType = std::string;
using NeighboursType = std::set<PhilosopherNameType>;
using NeighbourMapType = std::map<PhilosopherNameType, NeighboursType>;
std::vector<NeighbourMapType> table;
table.push_back(NeighbourMapType());
I mention this because you can likely still benefit from this in other places.
2
agreed. Along the same lines are the types that the STL gives you (vector::value_type iirc)
– sudo rm -rf slash
Mar 26 at 8:01
add a comment |
Though the other answers are correct, I will add that if you couldn't take that approach, you could have benefitted from declaring some type aliases to shorten that container type name.
I can of course only guess at the logical meaning of your containers, which is another thing that this fixes!
using PhilosopherNameType = std::string;
using NeighboursType = std::set<PhilosopherNameType>;
using NeighbourMapType = std::map<PhilosopherNameType, NeighboursType>;
std::vector<NeighbourMapType> table;
table.push_back(NeighbourMapType());
I mention this because you can likely still benefit from this in other places.
Though the other answers are correct, I will add that if you couldn't take that approach, you could have benefitted from declaring some type aliases to shorten that container type name.
I can of course only guess at the logical meaning of your containers, which is another thing that this fixes!
using PhilosopherNameType = std::string;
using NeighboursType = std::set<PhilosopherNameType>;
using NeighbourMapType = std::map<PhilosopherNameType, NeighboursType>;
std::vector<NeighbourMapType> table;
table.push_back(NeighbourMapType());
I mention this because you can likely still benefit from this in other places.
answered Mar 25 at 17:12


Lightness Races in OrbitLightness Races in Orbit
294k54477811
294k54477811
2
agreed. Along the same lines are the types that the STL gives you (vector::value_type iirc)
– sudo rm -rf slash
Mar 26 at 8:01
add a comment |
2
agreed. Along the same lines are the types that the STL gives you (vector::value_type iirc)
– sudo rm -rf slash
Mar 26 at 8:01
2
2
agreed. Along the same lines are the types that the STL gives you (vector::value_type iirc)
– sudo rm -rf slash
Mar 26 at 8:01
agreed. Along the same lines are the types that the STL gives you (vector::value_type iirc)
– sudo rm -rf slash
Mar 26 at 8:01
add a comment |
As a example:
vector<int> arr;
arr.push_back(1);
arr.push_back(2);
To use it, you should:
include
and also, either use std::vector
in your code or add
using std::vector;
or
using namespace std;
after the #include
line.
add a comment |
As a example:
vector<int> arr;
arr.push_back(1);
arr.push_back(2);
To use it, you should:
include
and also, either use std::vector
in your code or add
using std::vector;
or
using namespace std;
after the #include
line.
add a comment |
As a example:
vector<int> arr;
arr.push_back(1);
arr.push_back(2);
To use it, you should:
include
and also, either use std::vector
in your code or add
using std::vector;
or
using namespace std;
after the #include
line.
As a example:
vector<int> arr;
arr.push_back(1);
arr.push_back(2);
To use it, you should:
include
and also, either use std::vector
in your code or add
using std::vector;
or
using namespace std;
after the #include
line.
answered yesterday


Mr. SemicolonMr. Semicolon
5301422
5301422
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55332359%2fadding-empty-element-to-declared-container-without-declaring-type-of-element%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
gyL dYwhD,DKzl23Qg 7 ru6e JGPh8a8fJkLbqVBmdG1sCV,1RtBr5ayPpf0bTjEP,r0 RV l0SINgY6f5li XD,k4b7lRXj,Tkv1lN