How do I run multiple scripts within a script, even if one script fails
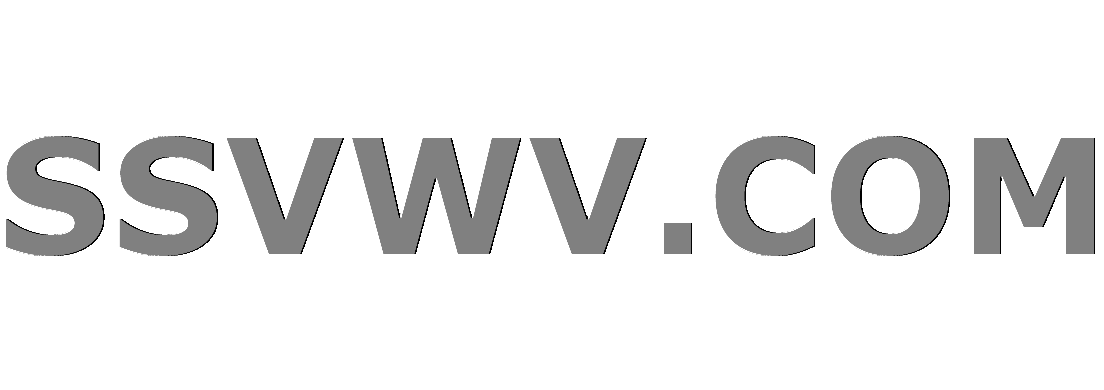
Multi tool use
I have a simple bash script that executes a number of other scripts …
#/bin/bash
…
./update_artifact1.sh $ARTIFACT_VERSION
./update_artifact2.sh $ARTIFACT_VERSION
./update_artifact3.sh $ARTIFACT_VERSION
The problem is, if the first command (or second) dies with an error, none of the subsequent commands are run. Is there a way I can run all three commands, save the exit status of each, and then return a successful exit status if all three execute successfully? The scripts don’t have to run concurrently.
bash bash-scripting exit-code
add a comment |
I have a simple bash script that executes a number of other scripts …
#/bin/bash
…
./update_artifact1.sh $ARTIFACT_VERSION
./update_artifact2.sh $ARTIFACT_VERSION
./update_artifact3.sh $ARTIFACT_VERSION
The problem is, if the first command (or second) dies with an error, none of the subsequent commands are run. Is there a way I can run all three commands, save the exit status of each, and then return a successful exit status if all three execute successfully? The scripts don’t have to run concurrently.
bash bash-scripting exit-code
2
What you show does not indicate that the main script should die. What is behind "…"?
– glenn jackman
Feb 21 '14 at 21:26
If the command "./update_artifact1.sh $ARTIFACT_VERSION" fails, the other two commands ("./update_artifact2.sh $ARTIFACT_VERSION" and "./update_artifact3.sh $ARTIFACT_VERSION") don't run. Do you understand?
– Dave
Feb 21 '14 at 21:45
3
yes I do. But if you trycd /unknown_dir; echo ok
, the echo command executed even though cd failed. Why do the 2nd and 3rd commands fail in your script? Do you haveset -e
turned on? Do the 2nd and 3rd commands rely on something the 1st provides? Is there any error output? You need to provide more information.
– glenn jackman
Feb 21 '14 at 22:17
add a comment |
I have a simple bash script that executes a number of other scripts …
#/bin/bash
…
./update_artifact1.sh $ARTIFACT_VERSION
./update_artifact2.sh $ARTIFACT_VERSION
./update_artifact3.sh $ARTIFACT_VERSION
The problem is, if the first command (or second) dies with an error, none of the subsequent commands are run. Is there a way I can run all three commands, save the exit status of each, and then return a successful exit status if all three execute successfully? The scripts don’t have to run concurrently.
bash bash-scripting exit-code
I have a simple bash script that executes a number of other scripts …
#/bin/bash
…
./update_artifact1.sh $ARTIFACT_VERSION
./update_artifact2.sh $ARTIFACT_VERSION
./update_artifact3.sh $ARTIFACT_VERSION
The problem is, if the first command (or second) dies with an error, none of the subsequent commands are run. Is there a way I can run all three commands, save the exit status of each, and then return a successful exit status if all three execute successfully? The scripts don’t have to run concurrently.
bash bash-scripting exit-code
bash bash-scripting exit-code
asked Feb 21 '14 at 21:15
DaveDave
2325
2325
2
What you show does not indicate that the main script should die. What is behind "…"?
– glenn jackman
Feb 21 '14 at 21:26
If the command "./update_artifact1.sh $ARTIFACT_VERSION" fails, the other two commands ("./update_artifact2.sh $ARTIFACT_VERSION" and "./update_artifact3.sh $ARTIFACT_VERSION") don't run. Do you understand?
– Dave
Feb 21 '14 at 21:45
3
yes I do. But if you trycd /unknown_dir; echo ok
, the echo command executed even though cd failed. Why do the 2nd and 3rd commands fail in your script? Do you haveset -e
turned on? Do the 2nd and 3rd commands rely on something the 1st provides? Is there any error output? You need to provide more information.
– glenn jackman
Feb 21 '14 at 22:17
add a comment |
2
What you show does not indicate that the main script should die. What is behind "…"?
– glenn jackman
Feb 21 '14 at 21:26
If the command "./update_artifact1.sh $ARTIFACT_VERSION" fails, the other two commands ("./update_artifact2.sh $ARTIFACT_VERSION" and "./update_artifact3.sh $ARTIFACT_VERSION") don't run. Do you understand?
– Dave
Feb 21 '14 at 21:45
3
yes I do. But if you trycd /unknown_dir; echo ok
, the echo command executed even though cd failed. Why do the 2nd and 3rd commands fail in your script? Do you haveset -e
turned on? Do the 2nd and 3rd commands rely on something the 1st provides? Is there any error output? You need to provide more information.
– glenn jackman
Feb 21 '14 at 22:17
2
2
What you show does not indicate that the main script should die. What is behind "…"?
– glenn jackman
Feb 21 '14 at 21:26
What you show does not indicate that the main script should die. What is behind "…"?
– glenn jackman
Feb 21 '14 at 21:26
If the command "./update_artifact1.sh $ARTIFACT_VERSION" fails, the other two commands ("./update_artifact2.sh $ARTIFACT_VERSION" and "./update_artifact3.sh $ARTIFACT_VERSION") don't run. Do you understand?
– Dave
Feb 21 '14 at 21:45
If the command "./update_artifact1.sh $ARTIFACT_VERSION" fails, the other two commands ("./update_artifact2.sh $ARTIFACT_VERSION" and "./update_artifact3.sh $ARTIFACT_VERSION") don't run. Do you understand?
– Dave
Feb 21 '14 at 21:45
3
3
yes I do. But if you try
cd /unknown_dir; echo ok
, the echo command executed even though cd failed. Why do the 2nd and 3rd commands fail in your script? Do you have set -e
turned on? Do the 2nd and 3rd commands rely on something the 1st provides? Is there any error output? You need to provide more information.– glenn jackman
Feb 21 '14 at 22:17
yes I do. But if you try
cd /unknown_dir; echo ok
, the echo command executed even though cd failed. Why do the 2nd and 3rd commands fail in your script? Do you have set -e
turned on? Do the 2nd and 3rd commands rely on something the 1st provides? Is there any error output? You need to provide more information.– glenn jackman
Feb 21 '14 at 22:17
add a comment |
1 Answer
1
active
oldest
votes
You can slightly modify your script in this way
#!/bin/bash
…
./update_artifact1.sh $ARTIFACT_VERSION
exit_state_1=$?
./update_artifact2.sh $ARTIFACT_VERSION
exit_state_2=$?
./update_artifact3.sh $ARTIFACT_VERSION
exit_state_3=$?
exit_state_all=$[ $exit_state_1 && $exit_state_2 && $exit_state_3 ]
exit $exit_state_all
Note:
- Each command return an exit code that
bash
stores in$?
. It is0
if there is no error, or a different number if there is an error.
After every line the value of$?
is updated so you need to store in a variable (in this case we usedexit_state_1
...exit_state_n
).
At the end you want that your script will return you only an error code with 0 if it is all ok and we realize it with$[ $a || $b || $c ]
.
Note that$[ 0|| 0 || 0 ]
is 0 meanwhile$[ $a || $b || $c ]
with at least one on$a,$b,$c
not equal to0
returns 1. - It is important the ! in the first line when you have to write
#!/bin/bash
to say to the system that is a script that have to be executed in abash
shell.
You may find interesting to read more about the shebang here.
- As noted in a comment too if you put
set -e
somewhere in your script before those line, the script will be interrupted at the first error without process the other line, and it will return an error code (the one of the last command executed).
add a comment |
Your Answer
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "3"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fsuperuser.com%2fquestions%2f720058%2fhow-do-i-run-multiple-scripts-within-a-script-even-if-one-script-fails%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can slightly modify your script in this way
#!/bin/bash
…
./update_artifact1.sh $ARTIFACT_VERSION
exit_state_1=$?
./update_artifact2.sh $ARTIFACT_VERSION
exit_state_2=$?
./update_artifact3.sh $ARTIFACT_VERSION
exit_state_3=$?
exit_state_all=$[ $exit_state_1 && $exit_state_2 && $exit_state_3 ]
exit $exit_state_all
Note:
- Each command return an exit code that
bash
stores in$?
. It is0
if there is no error, or a different number if there is an error.
After every line the value of$?
is updated so you need to store in a variable (in this case we usedexit_state_1
...exit_state_n
).
At the end you want that your script will return you only an error code with 0 if it is all ok and we realize it with$[ $a || $b || $c ]
.
Note that$[ 0|| 0 || 0 ]
is 0 meanwhile$[ $a || $b || $c ]
with at least one on$a,$b,$c
not equal to0
returns 1. - It is important the ! in the first line when you have to write
#!/bin/bash
to say to the system that is a script that have to be executed in abash
shell.
You may find interesting to read more about the shebang here.
- As noted in a comment too if you put
set -e
somewhere in your script before those line, the script will be interrupted at the first error without process the other line, and it will return an error code (the one of the last command executed).
add a comment |
You can slightly modify your script in this way
#!/bin/bash
…
./update_artifact1.sh $ARTIFACT_VERSION
exit_state_1=$?
./update_artifact2.sh $ARTIFACT_VERSION
exit_state_2=$?
./update_artifact3.sh $ARTIFACT_VERSION
exit_state_3=$?
exit_state_all=$[ $exit_state_1 && $exit_state_2 && $exit_state_3 ]
exit $exit_state_all
Note:
- Each command return an exit code that
bash
stores in$?
. It is0
if there is no error, or a different number if there is an error.
After every line the value of$?
is updated so you need to store in a variable (in this case we usedexit_state_1
...exit_state_n
).
At the end you want that your script will return you only an error code with 0 if it is all ok and we realize it with$[ $a || $b || $c ]
.
Note that$[ 0|| 0 || 0 ]
is 0 meanwhile$[ $a || $b || $c ]
with at least one on$a,$b,$c
not equal to0
returns 1. - It is important the ! in the first line when you have to write
#!/bin/bash
to say to the system that is a script that have to be executed in abash
shell.
You may find interesting to read more about the shebang here.
- As noted in a comment too if you put
set -e
somewhere in your script before those line, the script will be interrupted at the first error without process the other line, and it will return an error code (the one of the last command executed).
add a comment |
You can slightly modify your script in this way
#!/bin/bash
…
./update_artifact1.sh $ARTIFACT_VERSION
exit_state_1=$?
./update_artifact2.sh $ARTIFACT_VERSION
exit_state_2=$?
./update_artifact3.sh $ARTIFACT_VERSION
exit_state_3=$?
exit_state_all=$[ $exit_state_1 && $exit_state_2 && $exit_state_3 ]
exit $exit_state_all
Note:
- Each command return an exit code that
bash
stores in$?
. It is0
if there is no error, or a different number if there is an error.
After every line the value of$?
is updated so you need to store in a variable (in this case we usedexit_state_1
...exit_state_n
).
At the end you want that your script will return you only an error code with 0 if it is all ok and we realize it with$[ $a || $b || $c ]
.
Note that$[ 0|| 0 || 0 ]
is 0 meanwhile$[ $a || $b || $c ]
with at least one on$a,$b,$c
not equal to0
returns 1. - It is important the ! in the first line when you have to write
#!/bin/bash
to say to the system that is a script that have to be executed in abash
shell.
You may find interesting to read more about the shebang here.
- As noted in a comment too if you put
set -e
somewhere in your script before those line, the script will be interrupted at the first error without process the other line, and it will return an error code (the one of the last command executed).
You can slightly modify your script in this way
#!/bin/bash
…
./update_artifact1.sh $ARTIFACT_VERSION
exit_state_1=$?
./update_artifact2.sh $ARTIFACT_VERSION
exit_state_2=$?
./update_artifact3.sh $ARTIFACT_VERSION
exit_state_3=$?
exit_state_all=$[ $exit_state_1 && $exit_state_2 && $exit_state_3 ]
exit $exit_state_all
Note:
- Each command return an exit code that
bash
stores in$?
. It is0
if there is no error, or a different number if there is an error.
After every line the value of$?
is updated so you need to store in a variable (in this case we usedexit_state_1
...exit_state_n
).
At the end you want that your script will return you only an error code with 0 if it is all ok and we realize it with$[ $a || $b || $c ]
.
Note that$[ 0|| 0 || 0 ]
is 0 meanwhile$[ $a || $b || $c ]
with at least one on$a,$b,$c
not equal to0
returns 1. - It is important the ! in the first line when you have to write
#!/bin/bash
to say to the system that is a script that have to be executed in abash
shell.
You may find interesting to read more about the shebang here.
- As noted in a comment too if you put
set -e
somewhere in your script before those line, the script will be interrupted at the first error without process the other line, and it will return an error code (the one of the last command executed).
edited Jun 25 '14 at 15:21
answered Jun 25 '14 at 14:26
HasturHastur
13.2k53268
13.2k53268
add a comment |
add a comment |
Thanks for contributing an answer to Super User!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fsuperuser.com%2fquestions%2f720058%2fhow-do-i-run-multiple-scripts-within-a-script-even-if-one-script-fails%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
aCV,mMN265aDWA I8fLUsTuOz3,gFJwl1cIZ,zkBM8
2
What you show does not indicate that the main script should die. What is behind "…"?
– glenn jackman
Feb 21 '14 at 21:26
If the command "./update_artifact1.sh $ARTIFACT_VERSION" fails, the other two commands ("./update_artifact2.sh $ARTIFACT_VERSION" and "./update_artifact3.sh $ARTIFACT_VERSION") don't run. Do you understand?
– Dave
Feb 21 '14 at 21:45
3
yes I do. But if you try
cd /unknown_dir; echo ok
, the echo command executed even though cd failed. Why do the 2nd and 3rd commands fail in your script? Do you haveset -e
turned on? Do the 2nd and 3rd commands rely on something the 1st provides? Is there any error output? You need to provide more information.– glenn jackman
Feb 21 '14 at 22:17