Is there a way to add tshark output into a wx.ListCtrl?
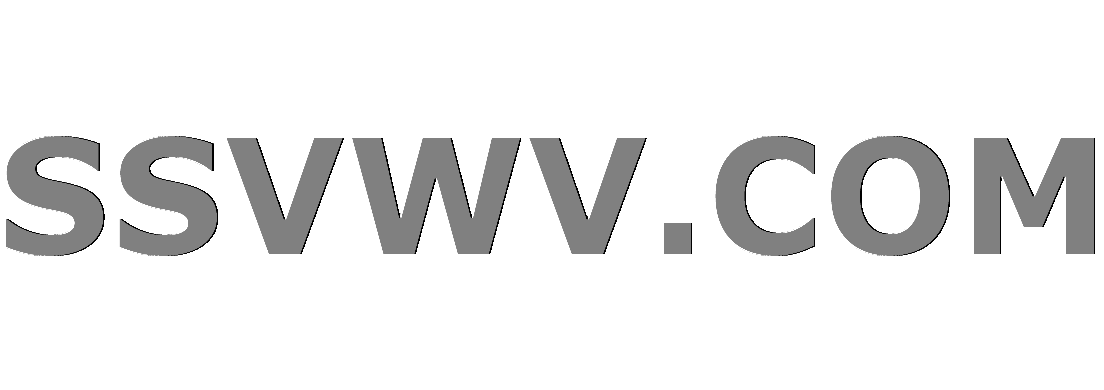
Multi tool use
currently i'm working on a GUI that analyzes a PCAP file. I'm a total beginner in Python/wxPython so I would really appreciate some friendly help!
I'm using tshark to read my PCAP file and wxPython4.0.1 for the GUI. The output of the tshark read is stored in a subprocess.popen(). From here on, I intend to show the output through a ListCtrl in the GUI so that it is more organized and readable. Is there a way to add the tshark output into the ListCtrl?
Here's the main part of my code:
import wx
import os
from subprocess import Popen, PIPE
import sys
class MyFrame(wx.Frame):
def __init__(self, *args, **kwds):
# begin wxGlade: MyFrame.__init__
kwds["style"] = kwds.get("style", 0) | wx.DEFAULT_FRAME_STYLE
wx.Frame.__init__(self, *args, **kwds)
self.SetSize((965, 600))
self.panel_1 = wx.Panel(self, wx.ID_ANY)
self.list_ctrl_1 = wx.ListCtrl(self.panel_1, wx.ID_ANY, style=wx.LC_HRULES | wx.LC_REPORT | wx.LC_SINGLE_SEL | wx.LC_VRULES)
# Menu Bar
self.frame_menubar = wx.MenuBar()
wxglade_tmp_menu = wx.Menu()
item = wxglade_tmp_menu.Append(wx.ID_ANY, "Upload PCAP", "")
self.Bind(wx.EVT_MENU, self.on_menu_upload, id=item.GetId())
self.frame_menubar.Append(wxglade_tmp_menu, "File")
self.SetMenuBar(self.frame_menubar)
# Menu Bar end
self.__set_properties()
self.__do_layout()
# end wxGlade
def __set_properties(self):
# begin wxGlade: MyFrame.__set_properties
self.SetTitle("Analysis")
self.list_ctrl_1.AppendColumn("Time", format=wx.LIST_FORMAT_LEFT, width=193)
self.list_ctrl_1.AppendColumn("#", format=wx.LIST_FORMAT_LEFT, width=185)
self.list_ctrl_1.AppendColumn("Source", format=wx.LIST_FORMAT_LEFT, width=193)
self.list_ctrl_1.AppendColumn("Destination", format=wx.LIST_FORMAT_LEFT, width=193)
self.list_ctrl_1.AppendColumn("Protocol", format=wx.LIST_FORMAT_LEFT, width=193)
# end wxGlade
def __do_layout(self):
# begin wxGlade: MyFrame.__do_layout
sizer_1 = wx.BoxSizer(wx.VERTICAL)
sizer_2 = wx.BoxSizer(wx.VERTICAL)
sizer_2.Add(self.list_ctrl_1, 1, wx.EXPAND, 0)
sizer_2.Add((0, 0), 0, 0, 0)
self.panel_1.SetSizer(sizer_2)
sizer_1.Add(self.panel_1, 1, wx.EXPAND, 0)
self.SetSizer(sizer_1)
self.Layout()
# end wxGlade
def on_menu_upload(self, event): # wxGlade: MyFrame.<event_handler>
openFileDialog = wx.FileDialog(self, "Open", "", "","*.pcap", #creates a filedialog that only allow user to select .pcap files
wx.FD_OPEN | wx.FD_FILE_MUST_EXIST)
if openFileDialog.ShowModal() == wx.ID_OK:
evidencePath = openFileDialog.GetPath() #get path of selected dd file
filename = os.path.basename(evidencePath)
cmd = ['tshark', '-r', filename]
process = Popen(cmd, stdout=PIPE, stderr=PIPE)
stdout, stderr = process.communicate()
"""self.list_ctrl_1.InsertStringItem(stdout)""" #insert the output into the ListCtrl
print("PCAP Uploaded")
else:
print("Error Occurred!")
# end of class MyFrame
python
migrated from superuser.com Dec 26 '18 at 9:30
This question came from our site for computer enthusiasts and power users.
add a comment |
currently i'm working on a GUI that analyzes a PCAP file. I'm a total beginner in Python/wxPython so I would really appreciate some friendly help!
I'm using tshark to read my PCAP file and wxPython4.0.1 for the GUI. The output of the tshark read is stored in a subprocess.popen(). From here on, I intend to show the output through a ListCtrl in the GUI so that it is more organized and readable. Is there a way to add the tshark output into the ListCtrl?
Here's the main part of my code:
import wx
import os
from subprocess import Popen, PIPE
import sys
class MyFrame(wx.Frame):
def __init__(self, *args, **kwds):
# begin wxGlade: MyFrame.__init__
kwds["style"] = kwds.get("style", 0) | wx.DEFAULT_FRAME_STYLE
wx.Frame.__init__(self, *args, **kwds)
self.SetSize((965, 600))
self.panel_1 = wx.Panel(self, wx.ID_ANY)
self.list_ctrl_1 = wx.ListCtrl(self.panel_1, wx.ID_ANY, style=wx.LC_HRULES | wx.LC_REPORT | wx.LC_SINGLE_SEL | wx.LC_VRULES)
# Menu Bar
self.frame_menubar = wx.MenuBar()
wxglade_tmp_menu = wx.Menu()
item = wxglade_tmp_menu.Append(wx.ID_ANY, "Upload PCAP", "")
self.Bind(wx.EVT_MENU, self.on_menu_upload, id=item.GetId())
self.frame_menubar.Append(wxglade_tmp_menu, "File")
self.SetMenuBar(self.frame_menubar)
# Menu Bar end
self.__set_properties()
self.__do_layout()
# end wxGlade
def __set_properties(self):
# begin wxGlade: MyFrame.__set_properties
self.SetTitle("Analysis")
self.list_ctrl_1.AppendColumn("Time", format=wx.LIST_FORMAT_LEFT, width=193)
self.list_ctrl_1.AppendColumn("#", format=wx.LIST_FORMAT_LEFT, width=185)
self.list_ctrl_1.AppendColumn("Source", format=wx.LIST_FORMAT_LEFT, width=193)
self.list_ctrl_1.AppendColumn("Destination", format=wx.LIST_FORMAT_LEFT, width=193)
self.list_ctrl_1.AppendColumn("Protocol", format=wx.LIST_FORMAT_LEFT, width=193)
# end wxGlade
def __do_layout(self):
# begin wxGlade: MyFrame.__do_layout
sizer_1 = wx.BoxSizer(wx.VERTICAL)
sizer_2 = wx.BoxSizer(wx.VERTICAL)
sizer_2.Add(self.list_ctrl_1, 1, wx.EXPAND, 0)
sizer_2.Add((0, 0), 0, 0, 0)
self.panel_1.SetSizer(sizer_2)
sizer_1.Add(self.panel_1, 1, wx.EXPAND, 0)
self.SetSizer(sizer_1)
self.Layout()
# end wxGlade
def on_menu_upload(self, event): # wxGlade: MyFrame.<event_handler>
openFileDialog = wx.FileDialog(self, "Open", "", "","*.pcap", #creates a filedialog that only allow user to select .pcap files
wx.FD_OPEN | wx.FD_FILE_MUST_EXIST)
if openFileDialog.ShowModal() == wx.ID_OK:
evidencePath = openFileDialog.GetPath() #get path of selected dd file
filename = os.path.basename(evidencePath)
cmd = ['tshark', '-r', filename]
process = Popen(cmd, stdout=PIPE, stderr=PIPE)
stdout, stderr = process.communicate()
"""self.list_ctrl_1.InsertStringItem(stdout)""" #insert the output into the ListCtrl
print("PCAP Uploaded")
else:
print("Error Occurred!")
# end of class MyFrame
python
migrated from superuser.com Dec 26 '18 at 9:30
This question came from our site for computer enthusiasts and power users.
You might have better luck using sharkd instead of tshark.
– Gerald Combs
Jan 2 at 19:13
add a comment |
currently i'm working on a GUI that analyzes a PCAP file. I'm a total beginner in Python/wxPython so I would really appreciate some friendly help!
I'm using tshark to read my PCAP file and wxPython4.0.1 for the GUI. The output of the tshark read is stored in a subprocess.popen(). From here on, I intend to show the output through a ListCtrl in the GUI so that it is more organized and readable. Is there a way to add the tshark output into the ListCtrl?
Here's the main part of my code:
import wx
import os
from subprocess import Popen, PIPE
import sys
class MyFrame(wx.Frame):
def __init__(self, *args, **kwds):
# begin wxGlade: MyFrame.__init__
kwds["style"] = kwds.get("style", 0) | wx.DEFAULT_FRAME_STYLE
wx.Frame.__init__(self, *args, **kwds)
self.SetSize((965, 600))
self.panel_1 = wx.Panel(self, wx.ID_ANY)
self.list_ctrl_1 = wx.ListCtrl(self.panel_1, wx.ID_ANY, style=wx.LC_HRULES | wx.LC_REPORT | wx.LC_SINGLE_SEL | wx.LC_VRULES)
# Menu Bar
self.frame_menubar = wx.MenuBar()
wxglade_tmp_menu = wx.Menu()
item = wxglade_tmp_menu.Append(wx.ID_ANY, "Upload PCAP", "")
self.Bind(wx.EVT_MENU, self.on_menu_upload, id=item.GetId())
self.frame_menubar.Append(wxglade_tmp_menu, "File")
self.SetMenuBar(self.frame_menubar)
# Menu Bar end
self.__set_properties()
self.__do_layout()
# end wxGlade
def __set_properties(self):
# begin wxGlade: MyFrame.__set_properties
self.SetTitle("Analysis")
self.list_ctrl_1.AppendColumn("Time", format=wx.LIST_FORMAT_LEFT, width=193)
self.list_ctrl_1.AppendColumn("#", format=wx.LIST_FORMAT_LEFT, width=185)
self.list_ctrl_1.AppendColumn("Source", format=wx.LIST_FORMAT_LEFT, width=193)
self.list_ctrl_1.AppendColumn("Destination", format=wx.LIST_FORMAT_LEFT, width=193)
self.list_ctrl_1.AppendColumn("Protocol", format=wx.LIST_FORMAT_LEFT, width=193)
# end wxGlade
def __do_layout(self):
# begin wxGlade: MyFrame.__do_layout
sizer_1 = wx.BoxSizer(wx.VERTICAL)
sizer_2 = wx.BoxSizer(wx.VERTICAL)
sizer_2.Add(self.list_ctrl_1, 1, wx.EXPAND, 0)
sizer_2.Add((0, 0), 0, 0, 0)
self.panel_1.SetSizer(sizer_2)
sizer_1.Add(self.panel_1, 1, wx.EXPAND, 0)
self.SetSizer(sizer_1)
self.Layout()
# end wxGlade
def on_menu_upload(self, event): # wxGlade: MyFrame.<event_handler>
openFileDialog = wx.FileDialog(self, "Open", "", "","*.pcap", #creates a filedialog that only allow user to select .pcap files
wx.FD_OPEN | wx.FD_FILE_MUST_EXIST)
if openFileDialog.ShowModal() == wx.ID_OK:
evidencePath = openFileDialog.GetPath() #get path of selected dd file
filename = os.path.basename(evidencePath)
cmd = ['tshark', '-r', filename]
process = Popen(cmd, stdout=PIPE, stderr=PIPE)
stdout, stderr = process.communicate()
"""self.list_ctrl_1.InsertStringItem(stdout)""" #insert the output into the ListCtrl
print("PCAP Uploaded")
else:
print("Error Occurred!")
# end of class MyFrame
python
currently i'm working on a GUI that analyzes a PCAP file. I'm a total beginner in Python/wxPython so I would really appreciate some friendly help!
I'm using tshark to read my PCAP file and wxPython4.0.1 for the GUI. The output of the tshark read is stored in a subprocess.popen(). From here on, I intend to show the output through a ListCtrl in the GUI so that it is more organized and readable. Is there a way to add the tshark output into the ListCtrl?
Here's the main part of my code:
import wx
import os
from subprocess import Popen, PIPE
import sys
class MyFrame(wx.Frame):
def __init__(self, *args, **kwds):
# begin wxGlade: MyFrame.__init__
kwds["style"] = kwds.get("style", 0) | wx.DEFAULT_FRAME_STYLE
wx.Frame.__init__(self, *args, **kwds)
self.SetSize((965, 600))
self.panel_1 = wx.Panel(self, wx.ID_ANY)
self.list_ctrl_1 = wx.ListCtrl(self.panel_1, wx.ID_ANY, style=wx.LC_HRULES | wx.LC_REPORT | wx.LC_SINGLE_SEL | wx.LC_VRULES)
# Menu Bar
self.frame_menubar = wx.MenuBar()
wxglade_tmp_menu = wx.Menu()
item = wxglade_tmp_menu.Append(wx.ID_ANY, "Upload PCAP", "")
self.Bind(wx.EVT_MENU, self.on_menu_upload, id=item.GetId())
self.frame_menubar.Append(wxglade_tmp_menu, "File")
self.SetMenuBar(self.frame_menubar)
# Menu Bar end
self.__set_properties()
self.__do_layout()
# end wxGlade
def __set_properties(self):
# begin wxGlade: MyFrame.__set_properties
self.SetTitle("Analysis")
self.list_ctrl_1.AppendColumn("Time", format=wx.LIST_FORMAT_LEFT, width=193)
self.list_ctrl_1.AppendColumn("#", format=wx.LIST_FORMAT_LEFT, width=185)
self.list_ctrl_1.AppendColumn("Source", format=wx.LIST_FORMAT_LEFT, width=193)
self.list_ctrl_1.AppendColumn("Destination", format=wx.LIST_FORMAT_LEFT, width=193)
self.list_ctrl_1.AppendColumn("Protocol", format=wx.LIST_FORMAT_LEFT, width=193)
# end wxGlade
def __do_layout(self):
# begin wxGlade: MyFrame.__do_layout
sizer_1 = wx.BoxSizer(wx.VERTICAL)
sizer_2 = wx.BoxSizer(wx.VERTICAL)
sizer_2.Add(self.list_ctrl_1, 1, wx.EXPAND, 0)
sizer_2.Add((0, 0), 0, 0, 0)
self.panel_1.SetSizer(sizer_2)
sizer_1.Add(self.panel_1, 1, wx.EXPAND, 0)
self.SetSizer(sizer_1)
self.Layout()
# end wxGlade
def on_menu_upload(self, event): # wxGlade: MyFrame.<event_handler>
openFileDialog = wx.FileDialog(self, "Open", "", "","*.pcap", #creates a filedialog that only allow user to select .pcap files
wx.FD_OPEN | wx.FD_FILE_MUST_EXIST)
if openFileDialog.ShowModal() == wx.ID_OK:
evidencePath = openFileDialog.GetPath() #get path of selected dd file
filename = os.path.basename(evidencePath)
cmd = ['tshark', '-r', filename]
process = Popen(cmd, stdout=PIPE, stderr=PIPE)
stdout, stderr = process.communicate()
"""self.list_ctrl_1.InsertStringItem(stdout)""" #insert the output into the ListCtrl
print("PCAP Uploaded")
else:
print("Error Occurred!")
# end of class MyFrame
python
python
asked Dec 26 '18 at 4:54
Lam
migrated from superuser.com Dec 26 '18 at 9:30
This question came from our site for computer enthusiasts and power users.
migrated from superuser.com Dec 26 '18 at 9:30
This question came from our site for computer enthusiasts and power users.
You might have better luck using sharkd instead of tshark.
– Gerald Combs
Jan 2 at 19:13
add a comment |
You might have better luck using sharkd instead of tshark.
– Gerald Combs
Jan 2 at 19:13
You might have better luck using sharkd instead of tshark.
– Gerald Combs
Jan 2 at 19:13
You might have better luck using sharkd instead of tshark.
– Gerald Combs
Jan 2 at 19:13
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53930052%2fis-there-a-way-to-add-tshark-output-into-a-wx-listctrl%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53930052%2fis-there-a-way-to-add-tshark-output-into-a-wx-listctrl%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
rr8FL2nFs7hG9FC,zJ4RFfYfdXKZa7 CuejH8ncPK92r8FAieRabwFJAK6Hm 9IJoNU6,s KoW15vWor,go7Zr,2WQL,AZ,x
You might have better luck using sharkd instead of tshark.
– Gerald Combs
Jan 2 at 19:13