Pass multiple values to pipe in Angular 6
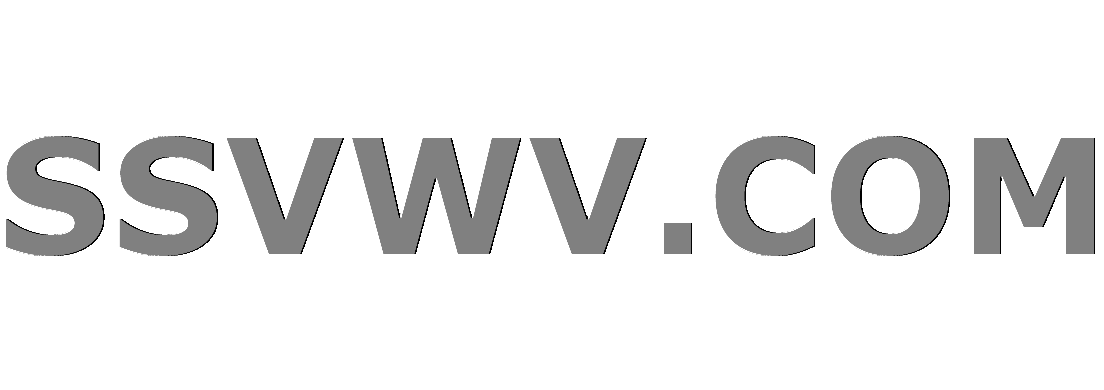
Multi tool use
I need to create a search form in Angular 6 with pipe and must pass multiple arguments to pipe .
nameSearch
, emailSearch
,roleSeach
, accountSearch
<tr *ngFor="let user of data | searchuser: nameSearch" ></tr>
and this my pipe :
@Pipe({
name: 'searchuser'
})
export class SearchuserPipe implements PipeTransform {
transform(users: IUser, nameSearch: string): IUser {
if(!users) return ;
if(!nameSearch) return users;
nameSearch=nameSearch.toLocaleLowerCase();
return users.filter(item=>
{
return item.desplayName.toLocaleLowerCase().includes(nameSearch)
});
}
please guide me how create pipe search with multi argument .
Edit :
transform(users: IUser, nameSearch: string ,eamilSearch:string,roleSearch:string): IUser {
if(!users) return ;
if(!nameSearch) return users;
if(!eamilSearch) return users;
if(!roleSearch) return users;
nameSearch=nameSearch.toLocaleLowerCase();
return users.filter(item=>
{
item.desplayName.toLocaleLowerCase().includes(nameSearch),
item.email.toLocaleLowerCase().includes(eamilSearch),
item.description.toLocaleLowerCase().includes(roleSearch)
});
}
angular angular6 angular-pipe
add a comment |
I need to create a search form in Angular 6 with pipe and must pass multiple arguments to pipe .
nameSearch
, emailSearch
,roleSeach
, accountSearch
<tr *ngFor="let user of data | searchuser: nameSearch" ></tr>
and this my pipe :
@Pipe({
name: 'searchuser'
})
export class SearchuserPipe implements PipeTransform {
transform(users: IUser, nameSearch: string): IUser {
if(!users) return ;
if(!nameSearch) return users;
nameSearch=nameSearch.toLocaleLowerCase();
return users.filter(item=>
{
return item.desplayName.toLocaleLowerCase().includes(nameSearch)
});
}
please guide me how create pipe search with multi argument .
Edit :
transform(users: IUser, nameSearch: string ,eamilSearch:string,roleSearch:string): IUser {
if(!users) return ;
if(!nameSearch) return users;
if(!eamilSearch) return users;
if(!roleSearch) return users;
nameSearch=nameSearch.toLocaleLowerCase();
return users.filter(item=>
{
item.desplayName.toLocaleLowerCase().includes(nameSearch),
item.email.toLocaleLowerCase().includes(eamilSearch),
item.description.toLocaleLowerCase().includes(roleSearch)
});
}
angular angular6 angular-pipe
1
Have you checked : angular.io/guide/pipes#custom-pipes ?
– Florian
Nov 30 '18 at 9:55
add a comment |
I need to create a search form in Angular 6 with pipe and must pass multiple arguments to pipe .
nameSearch
, emailSearch
,roleSeach
, accountSearch
<tr *ngFor="let user of data | searchuser: nameSearch" ></tr>
and this my pipe :
@Pipe({
name: 'searchuser'
})
export class SearchuserPipe implements PipeTransform {
transform(users: IUser, nameSearch: string): IUser {
if(!users) return ;
if(!nameSearch) return users;
nameSearch=nameSearch.toLocaleLowerCase();
return users.filter(item=>
{
return item.desplayName.toLocaleLowerCase().includes(nameSearch)
});
}
please guide me how create pipe search with multi argument .
Edit :
transform(users: IUser, nameSearch: string ,eamilSearch:string,roleSearch:string): IUser {
if(!users) return ;
if(!nameSearch) return users;
if(!eamilSearch) return users;
if(!roleSearch) return users;
nameSearch=nameSearch.toLocaleLowerCase();
return users.filter(item=>
{
item.desplayName.toLocaleLowerCase().includes(nameSearch),
item.email.toLocaleLowerCase().includes(eamilSearch),
item.description.toLocaleLowerCase().includes(roleSearch)
});
}
angular angular6 angular-pipe
I need to create a search form in Angular 6 with pipe and must pass multiple arguments to pipe .
nameSearch
, emailSearch
,roleSeach
, accountSearch
<tr *ngFor="let user of data | searchuser: nameSearch" ></tr>
and this my pipe :
@Pipe({
name: 'searchuser'
})
export class SearchuserPipe implements PipeTransform {
transform(users: IUser, nameSearch: string): IUser {
if(!users) return ;
if(!nameSearch) return users;
nameSearch=nameSearch.toLocaleLowerCase();
return users.filter(item=>
{
return item.desplayName.toLocaleLowerCase().includes(nameSearch)
});
}
please guide me how create pipe search with multi argument .
Edit :
transform(users: IUser, nameSearch: string ,eamilSearch:string,roleSearch:string): IUser {
if(!users) return ;
if(!nameSearch) return users;
if(!eamilSearch) return users;
if(!roleSearch) return users;
nameSearch=nameSearch.toLocaleLowerCase();
return users.filter(item=>
{
item.desplayName.toLocaleLowerCase().includes(nameSearch),
item.email.toLocaleLowerCase().includes(eamilSearch),
item.description.toLocaleLowerCase().includes(roleSearch)
});
}
angular angular6 angular-pipe
angular angular6 angular-pipe
edited Nov 30 '18 at 11:38
Mr-Programer
asked Nov 30 '18 at 9:48
Mr-ProgramerMr-Programer
847
847
1
Have you checked : angular.io/guide/pipes#custom-pipes ?
– Florian
Nov 30 '18 at 9:55
add a comment |
1
Have you checked : angular.io/guide/pipes#custom-pipes ?
– Florian
Nov 30 '18 at 9:55
1
1
Have you checked : angular.io/guide/pipes#custom-pipes ?
– Florian
Nov 30 '18 at 9:55
Have you checked : angular.io/guide/pipes#custom-pipes ?
– Florian
Nov 30 '18 at 9:55
add a comment |
2 Answers
2
active
oldest
votes
You can add more parameters to the transform
method that you'll have to implement in the pipe.
Make these parameters as optional so that you could use them as per your convenience.
Something like this:
import { Pipe, PipeTransform } from '@angular/core';
export interface IUser {
displayName: string;
name: string;
email: string;
role: string;
account: string;
description: string;
}
@Pipe({
name: 'searchUser'
})
export class SearchUserPipe implements PipeTransform {
transform(
users: IUser,
nameSearch?: string,
emailSearch?: string,
roleSearch?: string,
accountSearch?: string
): IUser {
if (!users) return ;
if (!nameSearch) return users;
nameSearch = nameSearch.toLocaleLowerCase();
users = [...users.filter(user => user.displayName.toLocaleLowerCase() === nameSearch)];
if (!emailSearch) return users;
emailSearch = emailSearch.toLocaleLowerCase();
users = [...users.filter(user => user.email.toLocaleLowerCase() === emailSearch)];
if (!roleSearch) return users;
roleSearch = roleSearch.toLocaleLowerCase();
users = [...users.filter(user => user.role.toLocaleLowerCase() === roleSearch)];
return users;
}
}
Now in your template, you can simply use this pipe
and pass arguments separated by a color(:
), something like this:
<input type="text" placeholder="name" [(ngModel)]="nameSearch">
<input type="text" placeholder="email" [(ngModel)]="emailSearch">
<input type="text" placeholder="role" [(ngModel)]="roleSearch">
<input type="text" placeholder="account" [(ngModel)]="accountSearch">
<table>
<tbody>
<tr *ngFor="let user of data | searchUser: nameSearch: emailSearch: roleSearch: accountSearch">
<td>
{{ user | json }}
</td>
</tr>
</tbody>
</table>
Here's also the Component Code:
import { Component } from '@angular/core';
@Component({...})
export class AppComponent {
nameSearch = '';
emailSearch = '';
roleSearch = '';
accountSearch = '';
data = [...];
}
Here's a Working Sample StackBlitz for your ref.
1
thanks when i using your code it not show me any list
– Mr-Programer
Nov 30 '18 at 10:57
i edit the question
– Mr-Programer
Nov 30 '18 at 11:00
@kianoush, could you please add some sample data as well to work with?
– SiddAjmera
Nov 30 '18 at 11:06
when i use one argument, it work , when i use 3 field justroleSearch
is work . i put the code in edit question
– Mr-Programer
Nov 30 '18 at 11:12
Yeah the overall idea is to just use the arguments separated by:
. But in case of static arguments wrap them around quotes. In case of arguments as I have as of now in the Updated answer and StackBlitz, you can use the properties as I've done.
– SiddAjmera
Nov 30 '18 at 11:13
|
show 3 more comments
It should be same way the you pass the single parameter with comma separation as follows,
export class SearchuserPipe implements PipeTransform {
transform(users: IUser, nameSearch:string, emailSearch:string, roleSearch:
string):IUser {
}
and in template,
<tr *ngFor="let user of data | searchuser: nameSearch : emailSearch : roleSearch" ></tr>
thanks but when i using this code it not work my pipt :transform(users: IUser, nameSearch: string ,eamilSearch:string,roleSearch:string): IUser { if(!users) return ; if(!nameSearch) return users; if(!eamilSearch) return users; if(!roleSearch) return users; nameSearch=nameSearch.toLocaleLowerCase(); return users.filter(item=> { item.desplayName.toLocaleLowerCase().includes(nameSearch), item.email.toLocaleLowerCase().includes(eamilSearch), item.description.toLocaleLowerCase().includes(roleSearch) });
– Mr-Programer
Nov 30 '18 at 10:57
i edit the question
– Mr-Programer
Nov 30 '18 at 11:00
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53555003%2fpass-multiple-values-to-pipe-in-angular-6%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can add more parameters to the transform
method that you'll have to implement in the pipe.
Make these parameters as optional so that you could use them as per your convenience.
Something like this:
import { Pipe, PipeTransform } from '@angular/core';
export interface IUser {
displayName: string;
name: string;
email: string;
role: string;
account: string;
description: string;
}
@Pipe({
name: 'searchUser'
})
export class SearchUserPipe implements PipeTransform {
transform(
users: IUser,
nameSearch?: string,
emailSearch?: string,
roleSearch?: string,
accountSearch?: string
): IUser {
if (!users) return ;
if (!nameSearch) return users;
nameSearch = nameSearch.toLocaleLowerCase();
users = [...users.filter(user => user.displayName.toLocaleLowerCase() === nameSearch)];
if (!emailSearch) return users;
emailSearch = emailSearch.toLocaleLowerCase();
users = [...users.filter(user => user.email.toLocaleLowerCase() === emailSearch)];
if (!roleSearch) return users;
roleSearch = roleSearch.toLocaleLowerCase();
users = [...users.filter(user => user.role.toLocaleLowerCase() === roleSearch)];
return users;
}
}
Now in your template, you can simply use this pipe
and pass arguments separated by a color(:
), something like this:
<input type="text" placeholder="name" [(ngModel)]="nameSearch">
<input type="text" placeholder="email" [(ngModel)]="emailSearch">
<input type="text" placeholder="role" [(ngModel)]="roleSearch">
<input type="text" placeholder="account" [(ngModel)]="accountSearch">
<table>
<tbody>
<tr *ngFor="let user of data | searchUser: nameSearch: emailSearch: roleSearch: accountSearch">
<td>
{{ user | json }}
</td>
</tr>
</tbody>
</table>
Here's also the Component Code:
import { Component } from '@angular/core';
@Component({...})
export class AppComponent {
nameSearch = '';
emailSearch = '';
roleSearch = '';
accountSearch = '';
data = [...];
}
Here's a Working Sample StackBlitz for your ref.
1
thanks when i using your code it not show me any list
– Mr-Programer
Nov 30 '18 at 10:57
i edit the question
– Mr-Programer
Nov 30 '18 at 11:00
@kianoush, could you please add some sample data as well to work with?
– SiddAjmera
Nov 30 '18 at 11:06
when i use one argument, it work , when i use 3 field justroleSearch
is work . i put the code in edit question
– Mr-Programer
Nov 30 '18 at 11:12
Yeah the overall idea is to just use the arguments separated by:
. But in case of static arguments wrap them around quotes. In case of arguments as I have as of now in the Updated answer and StackBlitz, you can use the properties as I've done.
– SiddAjmera
Nov 30 '18 at 11:13
|
show 3 more comments
You can add more parameters to the transform
method that you'll have to implement in the pipe.
Make these parameters as optional so that you could use them as per your convenience.
Something like this:
import { Pipe, PipeTransform } from '@angular/core';
export interface IUser {
displayName: string;
name: string;
email: string;
role: string;
account: string;
description: string;
}
@Pipe({
name: 'searchUser'
})
export class SearchUserPipe implements PipeTransform {
transform(
users: IUser,
nameSearch?: string,
emailSearch?: string,
roleSearch?: string,
accountSearch?: string
): IUser {
if (!users) return ;
if (!nameSearch) return users;
nameSearch = nameSearch.toLocaleLowerCase();
users = [...users.filter(user => user.displayName.toLocaleLowerCase() === nameSearch)];
if (!emailSearch) return users;
emailSearch = emailSearch.toLocaleLowerCase();
users = [...users.filter(user => user.email.toLocaleLowerCase() === emailSearch)];
if (!roleSearch) return users;
roleSearch = roleSearch.toLocaleLowerCase();
users = [...users.filter(user => user.role.toLocaleLowerCase() === roleSearch)];
return users;
}
}
Now in your template, you can simply use this pipe
and pass arguments separated by a color(:
), something like this:
<input type="text" placeholder="name" [(ngModel)]="nameSearch">
<input type="text" placeholder="email" [(ngModel)]="emailSearch">
<input type="text" placeholder="role" [(ngModel)]="roleSearch">
<input type="text" placeholder="account" [(ngModel)]="accountSearch">
<table>
<tbody>
<tr *ngFor="let user of data | searchUser: nameSearch: emailSearch: roleSearch: accountSearch">
<td>
{{ user | json }}
</td>
</tr>
</tbody>
</table>
Here's also the Component Code:
import { Component } from '@angular/core';
@Component({...})
export class AppComponent {
nameSearch = '';
emailSearch = '';
roleSearch = '';
accountSearch = '';
data = [...];
}
Here's a Working Sample StackBlitz for your ref.
1
thanks when i using your code it not show me any list
– Mr-Programer
Nov 30 '18 at 10:57
i edit the question
– Mr-Programer
Nov 30 '18 at 11:00
@kianoush, could you please add some sample data as well to work with?
– SiddAjmera
Nov 30 '18 at 11:06
when i use one argument, it work , when i use 3 field justroleSearch
is work . i put the code in edit question
– Mr-Programer
Nov 30 '18 at 11:12
Yeah the overall idea is to just use the arguments separated by:
. But in case of static arguments wrap them around quotes. In case of arguments as I have as of now in the Updated answer and StackBlitz, you can use the properties as I've done.
– SiddAjmera
Nov 30 '18 at 11:13
|
show 3 more comments
You can add more parameters to the transform
method that you'll have to implement in the pipe.
Make these parameters as optional so that you could use them as per your convenience.
Something like this:
import { Pipe, PipeTransform } from '@angular/core';
export interface IUser {
displayName: string;
name: string;
email: string;
role: string;
account: string;
description: string;
}
@Pipe({
name: 'searchUser'
})
export class SearchUserPipe implements PipeTransform {
transform(
users: IUser,
nameSearch?: string,
emailSearch?: string,
roleSearch?: string,
accountSearch?: string
): IUser {
if (!users) return ;
if (!nameSearch) return users;
nameSearch = nameSearch.toLocaleLowerCase();
users = [...users.filter(user => user.displayName.toLocaleLowerCase() === nameSearch)];
if (!emailSearch) return users;
emailSearch = emailSearch.toLocaleLowerCase();
users = [...users.filter(user => user.email.toLocaleLowerCase() === emailSearch)];
if (!roleSearch) return users;
roleSearch = roleSearch.toLocaleLowerCase();
users = [...users.filter(user => user.role.toLocaleLowerCase() === roleSearch)];
return users;
}
}
Now in your template, you can simply use this pipe
and pass arguments separated by a color(:
), something like this:
<input type="text" placeholder="name" [(ngModel)]="nameSearch">
<input type="text" placeholder="email" [(ngModel)]="emailSearch">
<input type="text" placeholder="role" [(ngModel)]="roleSearch">
<input type="text" placeholder="account" [(ngModel)]="accountSearch">
<table>
<tbody>
<tr *ngFor="let user of data | searchUser: nameSearch: emailSearch: roleSearch: accountSearch">
<td>
{{ user | json }}
</td>
</tr>
</tbody>
</table>
Here's also the Component Code:
import { Component } from '@angular/core';
@Component({...})
export class AppComponent {
nameSearch = '';
emailSearch = '';
roleSearch = '';
accountSearch = '';
data = [...];
}
Here's a Working Sample StackBlitz for your ref.
You can add more parameters to the transform
method that you'll have to implement in the pipe.
Make these parameters as optional so that you could use them as per your convenience.
Something like this:
import { Pipe, PipeTransform } from '@angular/core';
export interface IUser {
displayName: string;
name: string;
email: string;
role: string;
account: string;
description: string;
}
@Pipe({
name: 'searchUser'
})
export class SearchUserPipe implements PipeTransform {
transform(
users: IUser,
nameSearch?: string,
emailSearch?: string,
roleSearch?: string,
accountSearch?: string
): IUser {
if (!users) return ;
if (!nameSearch) return users;
nameSearch = nameSearch.toLocaleLowerCase();
users = [...users.filter(user => user.displayName.toLocaleLowerCase() === nameSearch)];
if (!emailSearch) return users;
emailSearch = emailSearch.toLocaleLowerCase();
users = [...users.filter(user => user.email.toLocaleLowerCase() === emailSearch)];
if (!roleSearch) return users;
roleSearch = roleSearch.toLocaleLowerCase();
users = [...users.filter(user => user.role.toLocaleLowerCase() === roleSearch)];
return users;
}
}
Now in your template, you can simply use this pipe
and pass arguments separated by a color(:
), something like this:
<input type="text" placeholder="name" [(ngModel)]="nameSearch">
<input type="text" placeholder="email" [(ngModel)]="emailSearch">
<input type="text" placeholder="role" [(ngModel)]="roleSearch">
<input type="text" placeholder="account" [(ngModel)]="accountSearch">
<table>
<tbody>
<tr *ngFor="let user of data | searchUser: nameSearch: emailSearch: roleSearch: accountSearch">
<td>
{{ user | json }}
</td>
</tr>
</tbody>
</table>
Here's also the Component Code:
import { Component } from '@angular/core';
@Component({...})
export class AppComponent {
nameSearch = '';
emailSearch = '';
roleSearch = '';
accountSearch = '';
data = [...];
}
Here's a Working Sample StackBlitz for your ref.
edited Nov 30 '18 at 11:25
answered Nov 30 '18 at 9:59
SiddAjmeraSiddAjmera
13.5k31137
13.5k31137
1
thanks when i using your code it not show me any list
– Mr-Programer
Nov 30 '18 at 10:57
i edit the question
– Mr-Programer
Nov 30 '18 at 11:00
@kianoush, could you please add some sample data as well to work with?
– SiddAjmera
Nov 30 '18 at 11:06
when i use one argument, it work , when i use 3 field justroleSearch
is work . i put the code in edit question
– Mr-Programer
Nov 30 '18 at 11:12
Yeah the overall idea is to just use the arguments separated by:
. But in case of static arguments wrap them around quotes. In case of arguments as I have as of now in the Updated answer and StackBlitz, you can use the properties as I've done.
– SiddAjmera
Nov 30 '18 at 11:13
|
show 3 more comments
1
thanks when i using your code it not show me any list
– Mr-Programer
Nov 30 '18 at 10:57
i edit the question
– Mr-Programer
Nov 30 '18 at 11:00
@kianoush, could you please add some sample data as well to work with?
– SiddAjmera
Nov 30 '18 at 11:06
when i use one argument, it work , when i use 3 field justroleSearch
is work . i put the code in edit question
– Mr-Programer
Nov 30 '18 at 11:12
Yeah the overall idea is to just use the arguments separated by:
. But in case of static arguments wrap them around quotes. In case of arguments as I have as of now in the Updated answer and StackBlitz, you can use the properties as I've done.
– SiddAjmera
Nov 30 '18 at 11:13
1
1
thanks when i using your code it not show me any list
– Mr-Programer
Nov 30 '18 at 10:57
thanks when i using your code it not show me any list
– Mr-Programer
Nov 30 '18 at 10:57
i edit the question
– Mr-Programer
Nov 30 '18 at 11:00
i edit the question
– Mr-Programer
Nov 30 '18 at 11:00
@kianoush, could you please add some sample data as well to work with?
– SiddAjmera
Nov 30 '18 at 11:06
@kianoush, could you please add some sample data as well to work with?
– SiddAjmera
Nov 30 '18 at 11:06
when i use one argument, it work , when i use 3 field just
roleSearch
is work . i put the code in edit question– Mr-Programer
Nov 30 '18 at 11:12
when i use one argument, it work , when i use 3 field just
roleSearch
is work . i put the code in edit question– Mr-Programer
Nov 30 '18 at 11:12
Yeah the overall idea is to just use the arguments separated by
:
. But in case of static arguments wrap them around quotes. In case of arguments as I have as of now in the Updated answer and StackBlitz, you can use the properties as I've done.– SiddAjmera
Nov 30 '18 at 11:13
Yeah the overall idea is to just use the arguments separated by
:
. But in case of static arguments wrap them around quotes. In case of arguments as I have as of now in the Updated answer and StackBlitz, you can use the properties as I've done.– SiddAjmera
Nov 30 '18 at 11:13
|
show 3 more comments
It should be same way the you pass the single parameter with comma separation as follows,
export class SearchuserPipe implements PipeTransform {
transform(users: IUser, nameSearch:string, emailSearch:string, roleSearch:
string):IUser {
}
and in template,
<tr *ngFor="let user of data | searchuser: nameSearch : emailSearch : roleSearch" ></tr>
thanks but when i using this code it not work my pipt :transform(users: IUser, nameSearch: string ,eamilSearch:string,roleSearch:string): IUser { if(!users) return ; if(!nameSearch) return users; if(!eamilSearch) return users; if(!roleSearch) return users; nameSearch=nameSearch.toLocaleLowerCase(); return users.filter(item=> { item.desplayName.toLocaleLowerCase().includes(nameSearch), item.email.toLocaleLowerCase().includes(eamilSearch), item.description.toLocaleLowerCase().includes(roleSearch) });
– Mr-Programer
Nov 30 '18 at 10:57
i edit the question
– Mr-Programer
Nov 30 '18 at 11:00
add a comment |
It should be same way the you pass the single parameter with comma separation as follows,
export class SearchuserPipe implements PipeTransform {
transform(users: IUser, nameSearch:string, emailSearch:string, roleSearch:
string):IUser {
}
and in template,
<tr *ngFor="let user of data | searchuser: nameSearch : emailSearch : roleSearch" ></tr>
thanks but when i using this code it not work my pipt :transform(users: IUser, nameSearch: string ,eamilSearch:string,roleSearch:string): IUser { if(!users) return ; if(!nameSearch) return users; if(!eamilSearch) return users; if(!roleSearch) return users; nameSearch=nameSearch.toLocaleLowerCase(); return users.filter(item=> { item.desplayName.toLocaleLowerCase().includes(nameSearch), item.email.toLocaleLowerCase().includes(eamilSearch), item.description.toLocaleLowerCase().includes(roleSearch) });
– Mr-Programer
Nov 30 '18 at 10:57
i edit the question
– Mr-Programer
Nov 30 '18 at 11:00
add a comment |
It should be same way the you pass the single parameter with comma separation as follows,
export class SearchuserPipe implements PipeTransform {
transform(users: IUser, nameSearch:string, emailSearch:string, roleSearch:
string):IUser {
}
and in template,
<tr *ngFor="let user of data | searchuser: nameSearch : emailSearch : roleSearch" ></tr>
It should be same way the you pass the single parameter with comma separation as follows,
export class SearchuserPipe implements PipeTransform {
transform(users: IUser, nameSearch:string, emailSearch:string, roleSearch:
string):IUser {
}
and in template,
<tr *ngFor="let user of data | searchuser: nameSearch : emailSearch : roleSearch" ></tr>
answered Nov 30 '18 at 9:52


SajeetharanSajeetharan
119k29166227
119k29166227
thanks but when i using this code it not work my pipt :transform(users: IUser, nameSearch: string ,eamilSearch:string,roleSearch:string): IUser { if(!users) return ; if(!nameSearch) return users; if(!eamilSearch) return users; if(!roleSearch) return users; nameSearch=nameSearch.toLocaleLowerCase(); return users.filter(item=> { item.desplayName.toLocaleLowerCase().includes(nameSearch), item.email.toLocaleLowerCase().includes(eamilSearch), item.description.toLocaleLowerCase().includes(roleSearch) });
– Mr-Programer
Nov 30 '18 at 10:57
i edit the question
– Mr-Programer
Nov 30 '18 at 11:00
add a comment |
thanks but when i using this code it not work my pipt :transform(users: IUser, nameSearch: string ,eamilSearch:string,roleSearch:string): IUser { if(!users) return ; if(!nameSearch) return users; if(!eamilSearch) return users; if(!roleSearch) return users; nameSearch=nameSearch.toLocaleLowerCase(); return users.filter(item=> { item.desplayName.toLocaleLowerCase().includes(nameSearch), item.email.toLocaleLowerCase().includes(eamilSearch), item.description.toLocaleLowerCase().includes(roleSearch) });
– Mr-Programer
Nov 30 '18 at 10:57
i edit the question
– Mr-Programer
Nov 30 '18 at 11:00
thanks but when i using this code it not work my pipt :
transform(users: IUser, nameSearch: string ,eamilSearch:string,roleSearch:string): IUser { if(!users) return ; if(!nameSearch) return users; if(!eamilSearch) return users; if(!roleSearch) return users; nameSearch=nameSearch.toLocaleLowerCase(); return users.filter(item=> { item.desplayName.toLocaleLowerCase().includes(nameSearch), item.email.toLocaleLowerCase().includes(eamilSearch), item.description.toLocaleLowerCase().includes(roleSearch) });
– Mr-Programer
Nov 30 '18 at 10:57
thanks but when i using this code it not work my pipt :
transform(users: IUser, nameSearch: string ,eamilSearch:string,roleSearch:string): IUser { if(!users) return ; if(!nameSearch) return users; if(!eamilSearch) return users; if(!roleSearch) return users; nameSearch=nameSearch.toLocaleLowerCase(); return users.filter(item=> { item.desplayName.toLocaleLowerCase().includes(nameSearch), item.email.toLocaleLowerCase().includes(eamilSearch), item.description.toLocaleLowerCase().includes(roleSearch) });
– Mr-Programer
Nov 30 '18 at 10:57
i edit the question
– Mr-Programer
Nov 30 '18 at 11:00
i edit the question
– Mr-Programer
Nov 30 '18 at 11:00
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53555003%2fpass-multiple-values-to-pipe-in-angular-6%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
b5DiO4nfvKwg3 IwEy5V08iEz9LYlJNAzJggMLD op PW,1
1
Have you checked : angular.io/guide/pipes#custom-pipes ?
– Florian
Nov 30 '18 at 9:55