Collect both matching and non-matching in one stream processing?
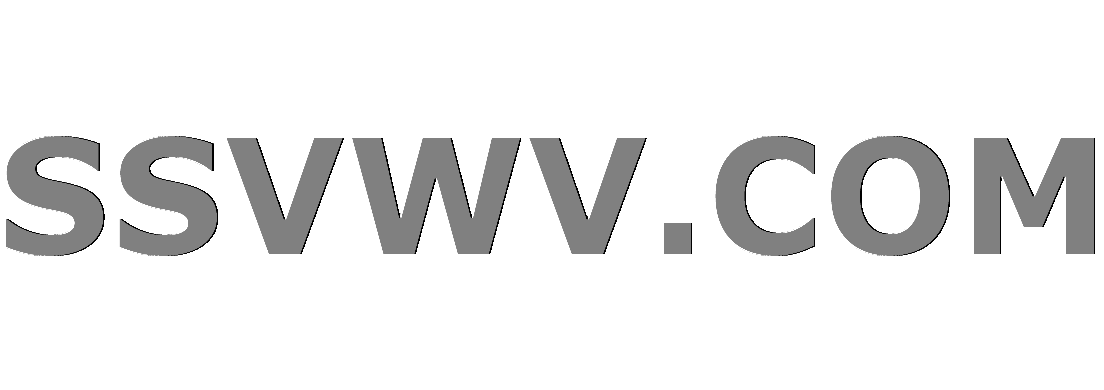
Multi tool use
up vote
17
down vote
favorite
Is there a way to collect both matching and not matching elements of stream in one processing?
Take this example:
final List<Integer> numbers = Arrays.asList( 1, 2, 3, 4, 5 );
final List<Integer> even = numbers.stream().filter( n -> n % 2 == 0 ).collect( Collectors.toList() );
final List<Integer> odd = numbers.stream().filter( n -> n % 2 != 0 ).collect( Collectors.toList() );
Is there a way to avoid running through the list of numbers twice? Something like "collector for matches and collector for no-matches"?
java java-stream
add a comment |
up vote
17
down vote
favorite
Is there a way to collect both matching and not matching elements of stream in one processing?
Take this example:
final List<Integer> numbers = Arrays.asList( 1, 2, 3, 4, 5 );
final List<Integer> even = numbers.stream().filter( n -> n % 2 == 0 ).collect( Collectors.toList() );
final List<Integer> odd = numbers.stream().filter( n -> n % 2 != 0 ).collect( Collectors.toList() );
Is there a way to avoid running through the list of numbers twice? Something like "collector for matches and collector for no-matches"?
java java-stream
5
SeeCollectors.partitioningBy
.
– Slaw
Nov 14 at 9:32
add a comment |
up vote
17
down vote
favorite
up vote
17
down vote
favorite
Is there a way to collect both matching and not matching elements of stream in one processing?
Take this example:
final List<Integer> numbers = Arrays.asList( 1, 2, 3, 4, 5 );
final List<Integer> even = numbers.stream().filter( n -> n % 2 == 0 ).collect( Collectors.toList() );
final List<Integer> odd = numbers.stream().filter( n -> n % 2 != 0 ).collect( Collectors.toList() );
Is there a way to avoid running through the list of numbers twice? Something like "collector for matches and collector for no-matches"?
java java-stream
Is there a way to collect both matching and not matching elements of stream in one processing?
Take this example:
final List<Integer> numbers = Arrays.asList( 1, 2, 3, 4, 5 );
final List<Integer> even = numbers.stream().filter( n -> n % 2 == 0 ).collect( Collectors.toList() );
final List<Integer> odd = numbers.stream().filter( n -> n % 2 != 0 ).collect( Collectors.toList() );
Is there a way to avoid running through the list of numbers twice? Something like "collector for matches and collector for no-matches"?
java java-stream
java java-stream
edited Nov 14 at 9:28


Oleksandr
7,72543467
7,72543467
asked Nov 14 at 9:27
Torgeist
1939
1939
5
SeeCollectors.partitioningBy
.
– Slaw
Nov 14 at 9:32
add a comment |
5
SeeCollectors.partitioningBy
.
– Slaw
Nov 14 at 9:32
5
5
See
Collectors.partitioningBy
.– Slaw
Nov 14 at 9:32
See
Collectors.partitioningBy
.– Slaw
Nov 14 at 9:32
add a comment |
2 Answers
2
active
oldest
votes
up vote
24
down vote
accepted
You may do it like so,
Map<Boolean, List<Integer>> oddAndEvenMap = numbers.stream()
.collect(Collectors.partitioningBy(n -> n % 2 == 0));
final List<Integer> even = oddAndEvenMap.get(true);
final List<Integer> odd = oddAndEvenMap.get(false);
Thank you very much, that was it - how could I miss this?! ;) Especially helpful is the partioningBy combined with another collector, like so:Map<Boolean,Map<Integer,Boolean>> infos = numbers.stream().collect( Collectors.partitioningBy( n -> n % 2 == 0, Collectors.toMap( n->n, n-> expensiveCalculation(n) ) ) );
– Torgeist
Nov 14 at 10:22
add a comment |
up vote
3
down vote
If you have more than 2 groups (instead of odd and even here using %2) for example to group ints in remainder classes %3 you can use a Function:
Function<Integer, Integer> fun = i -> i%3;
List<Integer> a = Arrays.asList(1,2,3,4,5,6,7,8,9,10);
Map<Integer, List<Integer>> collect = a.stream().collect(Collectors.groupingBy(fun));
System.out.println(collect);
//{0=[3, 6, 9], 1=[1, 4, 7, 10], 2=[2, 5, 8]}
Or imagine you have a list of strings which you want to group by starting char
instead of grouping matching and non-matching (for e.g. starts with a or not)
you could do something like :
Function<String, Character> fun = s -> s.charAt(0);
List<String> a = Arrays.asList("baz","buzz","azz","ayy","foo","doo");
Map<Character, List<String>> collect = a.stream().collect(Collectors.groupingBy(fun));
System.out.println(collect);
//{a=[azz, ayy], b=[baz, buzz], d=[doo], f=[foo]}
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
24
down vote
accepted
You may do it like so,
Map<Boolean, List<Integer>> oddAndEvenMap = numbers.stream()
.collect(Collectors.partitioningBy(n -> n % 2 == 0));
final List<Integer> even = oddAndEvenMap.get(true);
final List<Integer> odd = oddAndEvenMap.get(false);
Thank you very much, that was it - how could I miss this?! ;) Especially helpful is the partioningBy combined with another collector, like so:Map<Boolean,Map<Integer,Boolean>> infos = numbers.stream().collect( Collectors.partitioningBy( n -> n % 2 == 0, Collectors.toMap( n->n, n-> expensiveCalculation(n) ) ) );
– Torgeist
Nov 14 at 10:22
add a comment |
up vote
24
down vote
accepted
You may do it like so,
Map<Boolean, List<Integer>> oddAndEvenMap = numbers.stream()
.collect(Collectors.partitioningBy(n -> n % 2 == 0));
final List<Integer> even = oddAndEvenMap.get(true);
final List<Integer> odd = oddAndEvenMap.get(false);
Thank you very much, that was it - how could I miss this?! ;) Especially helpful is the partioningBy combined with another collector, like so:Map<Boolean,Map<Integer,Boolean>> infos = numbers.stream().collect( Collectors.partitioningBy( n -> n % 2 == 0, Collectors.toMap( n->n, n-> expensiveCalculation(n) ) ) );
– Torgeist
Nov 14 at 10:22
add a comment |
up vote
24
down vote
accepted
up vote
24
down vote
accepted
You may do it like so,
Map<Boolean, List<Integer>> oddAndEvenMap = numbers.stream()
.collect(Collectors.partitioningBy(n -> n % 2 == 0));
final List<Integer> even = oddAndEvenMap.get(true);
final List<Integer> odd = oddAndEvenMap.get(false);
You may do it like so,
Map<Boolean, List<Integer>> oddAndEvenMap = numbers.stream()
.collect(Collectors.partitioningBy(n -> n % 2 == 0));
final List<Integer> even = oddAndEvenMap.get(true);
final List<Integer> odd = oddAndEvenMap.get(false);
answered Nov 14 at 9:31


Ravindra Ranwala
7,56331332
7,56331332
Thank you very much, that was it - how could I miss this?! ;) Especially helpful is the partioningBy combined with another collector, like so:Map<Boolean,Map<Integer,Boolean>> infos = numbers.stream().collect( Collectors.partitioningBy( n -> n % 2 == 0, Collectors.toMap( n->n, n-> expensiveCalculation(n) ) ) );
– Torgeist
Nov 14 at 10:22
add a comment |
Thank you very much, that was it - how could I miss this?! ;) Especially helpful is the partioningBy combined with another collector, like so:Map<Boolean,Map<Integer,Boolean>> infos = numbers.stream().collect( Collectors.partitioningBy( n -> n % 2 == 0, Collectors.toMap( n->n, n-> expensiveCalculation(n) ) ) );
– Torgeist
Nov 14 at 10:22
Thank you very much, that was it - how could I miss this?! ;) Especially helpful is the partioningBy combined with another collector, like so:
Map<Boolean,Map<Integer,Boolean>> infos = numbers.stream().collect( Collectors.partitioningBy( n -> n % 2 == 0, Collectors.toMap( n->n, n-> expensiveCalculation(n) ) ) );
– Torgeist
Nov 14 at 10:22
Thank you very much, that was it - how could I miss this?! ;) Especially helpful is the partioningBy combined with another collector, like so:
Map<Boolean,Map<Integer,Boolean>> infos = numbers.stream().collect( Collectors.partitioningBy( n -> n % 2 == 0, Collectors.toMap( n->n, n-> expensiveCalculation(n) ) ) );
– Torgeist
Nov 14 at 10:22
add a comment |
up vote
3
down vote
If you have more than 2 groups (instead of odd and even here using %2) for example to group ints in remainder classes %3 you can use a Function:
Function<Integer, Integer> fun = i -> i%3;
List<Integer> a = Arrays.asList(1,2,3,4,5,6,7,8,9,10);
Map<Integer, List<Integer>> collect = a.stream().collect(Collectors.groupingBy(fun));
System.out.println(collect);
//{0=[3, 6, 9], 1=[1, 4, 7, 10], 2=[2, 5, 8]}
Or imagine you have a list of strings which you want to group by starting char
instead of grouping matching and non-matching (for e.g. starts with a or not)
you could do something like :
Function<String, Character> fun = s -> s.charAt(0);
List<String> a = Arrays.asList("baz","buzz","azz","ayy","foo","doo");
Map<Character, List<String>> collect = a.stream().collect(Collectors.groupingBy(fun));
System.out.println(collect);
//{a=[azz, ayy], b=[baz, buzz], d=[doo], f=[foo]}
add a comment |
up vote
3
down vote
If you have more than 2 groups (instead of odd and even here using %2) for example to group ints in remainder classes %3 you can use a Function:
Function<Integer, Integer> fun = i -> i%3;
List<Integer> a = Arrays.asList(1,2,3,4,5,6,7,8,9,10);
Map<Integer, List<Integer>> collect = a.stream().collect(Collectors.groupingBy(fun));
System.out.println(collect);
//{0=[3, 6, 9], 1=[1, 4, 7, 10], 2=[2, 5, 8]}
Or imagine you have a list of strings which you want to group by starting char
instead of grouping matching and non-matching (for e.g. starts with a or not)
you could do something like :
Function<String, Character> fun = s -> s.charAt(0);
List<String> a = Arrays.asList("baz","buzz","azz","ayy","foo","doo");
Map<Character, List<String>> collect = a.stream().collect(Collectors.groupingBy(fun));
System.out.println(collect);
//{a=[azz, ayy], b=[baz, buzz], d=[doo], f=[foo]}
add a comment |
up vote
3
down vote
up vote
3
down vote
If you have more than 2 groups (instead of odd and even here using %2) for example to group ints in remainder classes %3 you can use a Function:
Function<Integer, Integer> fun = i -> i%3;
List<Integer> a = Arrays.asList(1,2,3,4,5,6,7,8,9,10);
Map<Integer, List<Integer>> collect = a.stream().collect(Collectors.groupingBy(fun));
System.out.println(collect);
//{0=[3, 6, 9], 1=[1, 4, 7, 10], 2=[2, 5, 8]}
Or imagine you have a list of strings which you want to group by starting char
instead of grouping matching and non-matching (for e.g. starts with a or not)
you could do something like :
Function<String, Character> fun = s -> s.charAt(0);
List<String> a = Arrays.asList("baz","buzz","azz","ayy","foo","doo");
Map<Character, List<String>> collect = a.stream().collect(Collectors.groupingBy(fun));
System.out.println(collect);
//{a=[azz, ayy], b=[baz, buzz], d=[doo], f=[foo]}
If you have more than 2 groups (instead of odd and even here using %2) for example to group ints in remainder classes %3 you can use a Function:
Function<Integer, Integer> fun = i -> i%3;
List<Integer> a = Arrays.asList(1,2,3,4,5,6,7,8,9,10);
Map<Integer, List<Integer>> collect = a.stream().collect(Collectors.groupingBy(fun));
System.out.println(collect);
//{0=[3, 6, 9], 1=[1, 4, 7, 10], 2=[2, 5, 8]}
Or imagine you have a list of strings which you want to group by starting char
instead of grouping matching and non-matching (for e.g. starts with a or not)
you could do something like :
Function<String, Character> fun = s -> s.charAt(0);
List<String> a = Arrays.asList("baz","buzz","azz","ayy","foo","doo");
Map<Character, List<String>> collect = a.stream().collect(Collectors.groupingBy(fun));
System.out.println(collect);
//{a=[azz, ayy], b=[baz, buzz], d=[doo], f=[foo]}
edited Nov 14 at 10:51
answered Nov 14 at 10:33


Eritrean
2,8341814
2,8341814
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53296841%2fcollect-both-matching-and-non-matching-in-one-stream-processing%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
H9U3aa7Zjqq9,ilFENfeWgY9,5lZ4J0Q1a47p4xHx0NsETCt7XF210SU4FHQ CN8lbH n2clI 2jo,d7z,iwoPVUafow7SjxzpIyo1S G
5
See
Collectors.partitioningBy
.– Slaw
Nov 14 at 9:32